Recommanded Books |
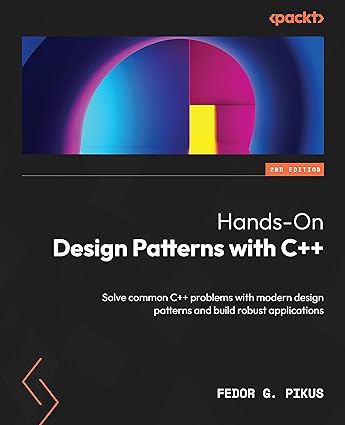 | Title | Hands-On Design Patterns with C++: Solve common C++ problems with modern design patterns and build robust applications |
ISBN | 978-1-804-61155-5 |
Author | Fedor G. Pikus |
Year | 2023 |
Publisher | Packtpub |
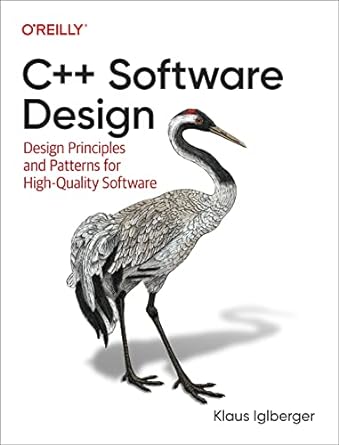 | Title | C++ Software Design: Design Principles and Patterns for High-Quality Software |
ISBN | 978-1-098-11316-2 |
Author | Klaus Iglberger |
Year | 2022 |
Publisher | O'Reilly Media |
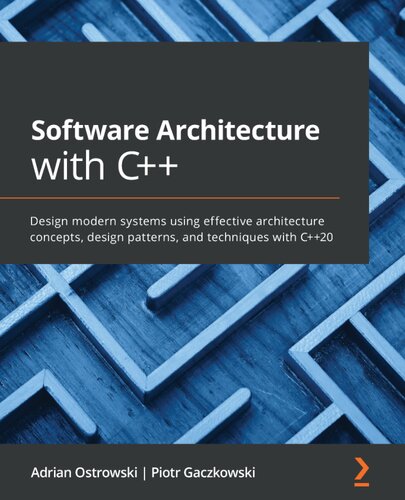 | Title | Software Architecture with C++: Design modern systems using effective architecture concepts, design patterns, and techniques with C++20 |
ISBN | 978-1-838-55459-0 |
Author | Adrian Ostrowski, Piotr Gaczkowski |
Year | 2021 |
Publisher | Packt |
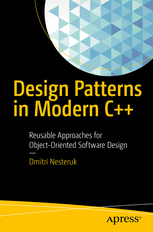 | Title | Design Patterns in Modern C++: Reusable Approaches for Object-oriented Software Design |
ISBN | 978-1-484-23603-1 |
Author | Dmitri Nesteruk |
Year | 2018 |
Publisher | Apress |