Recommanded Books |
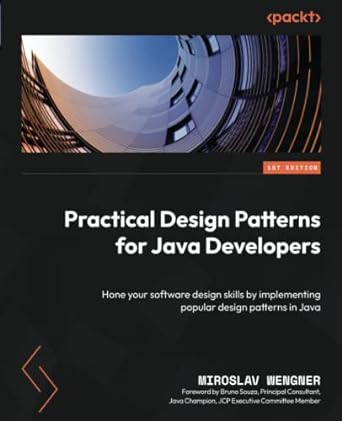 | Title | Practical Design Patterns for Java Developers: Hone your software design skills by implementing popular design patterns in Java |
ISBN | 978-1-804-61467-9 |
Author | Miroslav Wengner |
Year | 2023 |
Publisher | Packt Publishing |
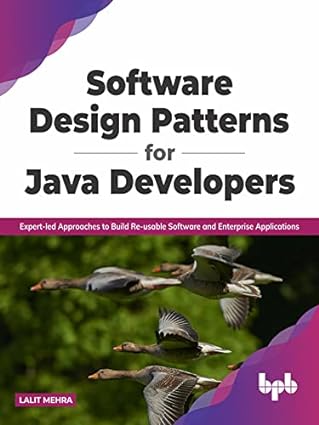 | Title | Software Design Patterns for Java Developers |
ISBN | B09MS4SH59 |
Author | Mehra, Lalit |
Year | 2023 |
Publisher | BPB Publications |
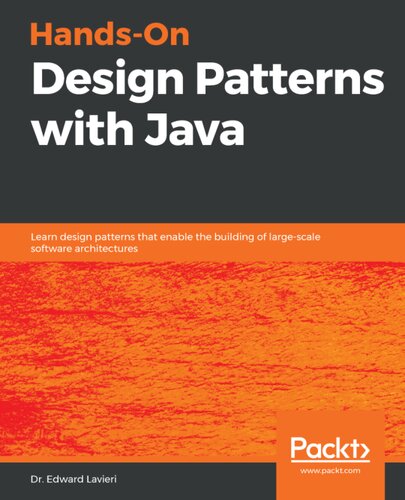 | Title | Hands-On Design Patterns with Java: Learn Design Patterns That Enable the Building of Large-Scale Software Architectures |
ISBN | 978-1-789-80977-0 |
Author | Edward Lavieri |
Year | 2020 |
Publisher | Packt |
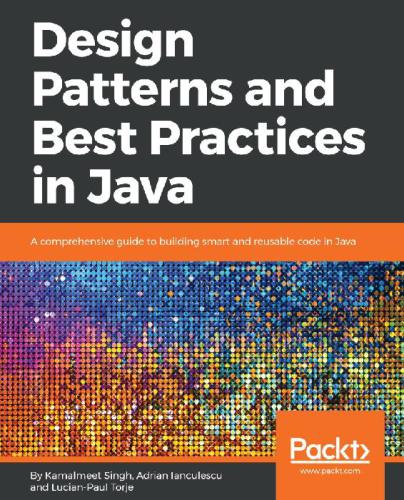 | Title | Design Patterns and Best Practices in Java: A comprehensive guide to building smart and reusable code in Java |
ISBN | 978-1-786-46359-3 |
Author | Lucian-Paul Torje & Adrian Ianculescu & Kamalmeet Singh [Lucian-Paul Torje] |
Year | 2018 |
Publisher | Packt Publishing |